In this tutorial you will learn how to use ESP8266 ESP-12E wifi module with Arduino IDE to blink a LED. This is basically a Hello World tutorial with ESP8266 using Arduino IDE. We are using ESP8266-12E version but other ESP8266 should also work.
In order to complete this tutorial we will first show, explain and configure the hardware and later on we will install the required ESP8266 board and library support. We will do hardware configuration first because when we configure the Arduino IDE to run program in the module, we need to specify the COM port that the USB to Serial converter is using to communicate with the ESP8266-12E.
Materials Required
What you need in this tutorial are the followings:
Hardware Aspects & Configuration
ESP8266(ESP-12E)
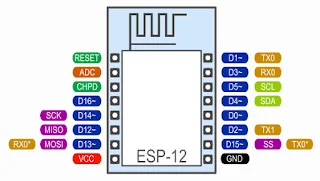
Wiring Diagram for Programming Circuit of ESP8266 ESP-12E & LED blink
The schematic diagram for connecting ESP8826/ESP-12E is as shown below.
The breadboard wiring diagram below shows how to connect ESP8266 ESP-12E with its supporting components, LED, power supply and USB to serial adaptor.
The supply voltage must be +3.3V on the power supply rails. The reset pin(RST), the enable pin(EN) and the GPIO0 pin of ESP8266-12E are pulled high to +3.3V using 10KOhm resistors. The GPIO15 is pulled low to ground using 10KOhm resistor. A 100nF capacitor is placed between VCC and ground pin of the ESP8266 module. Two push button switches are used to pull down the reset(RST) pin and GPIO0 pin. The TxD0 of the ESP8266 is connected to USB to serial device Rx pin and the and RxD0 of the ESP8266 is connected to the Tx pins. The ground pin of ESP8266(or breadboard) should be connected to the ground pin of the USB to Serial device. The Vcc pin of the ESP8266-12E should be connected to +3.3V. A LED with its positive terminal is connected GPIO4 pin and negative terminal to ground.
On actual breadboard it looks like this.
For identification purpose:
Programming the ESP8266 ESP-12E module
The buttons connected to Reset and GPIO0 are used for manual programming the ESP8266 ESP-12E wifi module. Manually means that we have to press the buttons to put the wifi module into USART programming mode to flash the code from the Arduino IDE to the memory of the ESP8266 module. In auto programming used in various ESP8266 delveopment boards such as NodeMCU or using FTDI programmer there are extra pins- DTR(Data Transmit Ready) and RTS(Request To Send), which are used to auto program the module. We are using simple USB to Serial adaptor which does not have the DTR and RTS pins so we have to manually put the Wifi module into programming mode.
To manually program the ESP8266 ESP12-E module, before uploading code, you have to follow certain sequence of pressing the two buttons which is outlined below.
It is important that whichever button you first first, during release you have release the Reset button first and then the USART mode button afterwards. To know whether you are in usart programming mode or not, you can use the GPIO16 and GPIO2. When in the USART programming mode, the the GPIO16 and GPIO2 will be high.
Software Aspects & Configuration
Installation ESP8266 Board & Libaries
1. Installation of Arduino core for ESP8266 WiFi chip
ESP8266 can be programmed using Arduino IDE using Arduino programming language. For this however we must install supporting board packages and libraries. These files can be found in the following link.
https://github.com/esp8266/Arduino
To install the board package and libraries open Arduino IDE > File > Preferences and copy paste the following link in the Additional Boards Manager URLs field.
https://arduino.esp8266.com/stable/package_esp8266com_index.json
Click OK and close the Preference window.
Next go to the Tools> Boards:"whatever board you using" > Board Manager... as shown below.
From there scroll down and find the esp8266 by ESP8266 community and install it as shown below,
2. Programming LED blink
After installing the board we have to specify the board and port number where the USB to Serial device is connected.
First specify the board by going to Tools> Boards:"whatever board you were using" and then selecting Generic ESP8266 Module.
Next check the COM port. Go to Tools > Port:"port number whichever you were using" and select the COM port to which your USB to Serial adapter is connected. As shown below, for example, in our case the USB to Serial adapter is connected to connected to COM 14 so we have selected 14.
If you don't know to which COM port your USB to serial is connected to, then right click on Computer from the start menu and then go to Device Manager. From Device manager Ports(COM & LPT) section and find your USB serial adapter port number. An example is shown below.
For LED blink we will use the GPIO4 pin so we configure the ESP8266 Builtin LED as 4 from the Tools menu as shown below.
For testing purpose aka "Hello World" we will use an example Blink sketch. For this go to File > Examples > ESP8266 > Blink as shown below.
You should see the the led blink script/sketch. We will change the default delay of 1000 microseconds and 2000 microseconds to 500 microseconds. Save the sketch in some folder. The led blink script code is shown below.
In the above program LED_BUILTIN is 4 or GPIO4 which we had set earlier.
3. Uploading LED blink code to ESP8266
Now its time for compiling the script and uploading it to ESP8266. Before uploading, press both the reset button or usart programming buttons(the order does not matter). Then first release the Reset button and programming button afterwards.
Now press the upload button in the Arduino IDE. The code will be compiled and uploaded to the ESP8266 wifi module. If everything went ok then you should see the LED connected to GPIO4 blinking with delay of 500 microseconds.
Video demonstration
The following video demonstrates the LED blinking with ESP8266 ESP12-E with Arduino IDE.
can you share a nodemcu library to download please?