In robotics and mechatronics projects, servo motors play a crucial role, and motor shields are valuable tools for running various types of motors. This tutorial will guide you through the process of controlling a Hobby Servo motor using the L293D Motor Shield and Arduino.
Servo Motor
A standard servo motor consists of three pins: ground, +5V, and a signal pin. The image below illustrates a simple Hobby Servo.
The L293D motor shield provides two ports, Servo Motor 1 and Servo Motor 2, for connecting two servo motors. Along with the power supply pins (Vcc and GND), the signal pins for Servo Motor 1 and Servo Motor 2 are connected to pins 9 and 10, respectively, on the Arduino UNO. Attaching the shield to the Arduino UNO establishes the connection between the servo signal pins and these designated Arduino pins.
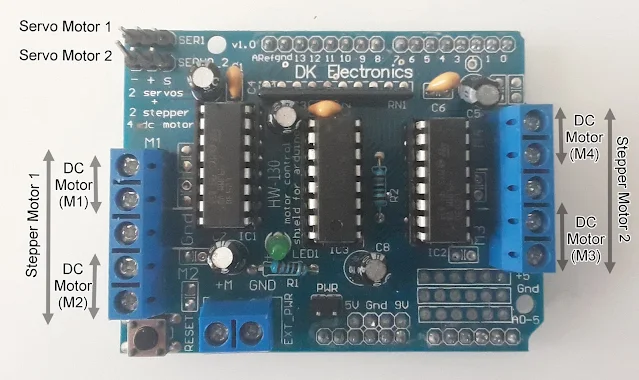
Interfacing the Servo Motor with L293D Motor Shield
Interfacing a servo motor with the L293D motor shield is a straightforward process. Simply connect the three pins of the servo motor to either the Servo Motor 1 or Servo Motor 2 labeled ports on the shield. In the example picture below, a servo is connected to the Servo Motor 1 port.
Programming Arduino for Servo Motor Control using Motor Shield
To program the servo motor, we can utilize the Servo library that comes with the Arduino IDE installation. Unlike controlling stepper or DC motors, the Adafruit motor library is unnecessary.
Here are the steps to program the servo motor:
Include the Servo library at the top of the sketch or program:
#include <Servo.h>
Create a Servo object using the Servo class:
Servo myservo;
Utilize the available methods:
Depending on the desired functionality, different methods are available. The attach() method is mandatory and should be declared in the setup() function within the program code.
a. Attach the Servo Object:
myservo.attach(9);
The attach() method accepts three parameters. In the above example, the first parameter is the pin number (9). The other two parameters are optional and represent the minimum and maximum pulse width in microseconds corresponding to the 0-degree and 180-degree angles, respectively. If not specified, default values of 544 and 2400 microseconds are used.
b. Detach the Servo Motor:
myservo.detach()
The detach() method disconnects the servo motor object from the PWM pin to which it is connected. Once detached, the PWM pin becomes available for other purposes.
c. Check Attachment:
myservo.attached()
The attached() method determines whether the servo object is attached to any pins. It returns TRUE or FALSE based on whether the servo object is attached or not.
d. Control Servo Position:
myservo.write(80)
The write() method adjusts the servo shaft's position by providing an angle value. In the given example, an angle of 80 degrees is specified, causing the servo to rotate accordingly. The angle value can range from 0 to 180.
e. Control Servo Position in Microseconds:
myservo.writeMicroseconds(1000)
The writeMicroseconds() method rotates the servo motor shaft to a specified orientation using the provided microseconds value. In the given example, 1000 microseconds are specified, resulting in the motor shaft rotating to the corresponding position. For a standard servo, 1000 microseconds, 1500 microseconds, and 2000 microseconds correspond to fully counter-clockwise, midpoint, and fully clockwise rotation angles, respectively.
f. Read Current Servo Angle:
servo.read()
The read() method returns the current angle value of the servo, which is the last value passed to the write() method.
Example Program Code for Servo Control using Motor Shield and Arduino:
Below is an example code that demonstrates how to rotate a servo motor from 0 to 180 degrees and then back to 0 degrees:
#include <Servo.h>
Servo myservo; // create a servo object to control a servo
const int servoPin = 10;
int pos = 0; // variable to store the servo position
void setup(){
// attach the servo on pin 10 to the servo object
myservo.attach(servoPin);
}
void loop(){
// sweep from 0 degrees to 180 degrees
for(pos = 0; pos <= 180; pos += 1){
myservo.write(pos);
delay(15);
}
// sweep from 180 degrees to 0 degrees
for(pos = 180; pos >= 0; pos -= 1){
myservo.write(pos);
delay(15);
}
}
Video Demonstration
The following video demonstrates the control of a servo motor using the Motor Shield and Arduino.
Conclusion
In this tutorial, we explored how to interface a Hobby Servo motor with the L293D Motor Shield and control it using an Arduino. By following the steps provided, you can easily program the servo motor using the Servo library and create various servo motor control functionalities. The Motor Shield simplifies the connection and provides a convenient platform for integrating servo motors into your robotics and mechatronics projects.
References
[1] control a DC motor using L293D Motor Shield
[2] control a Stepper Motor using L293D Motor Shield.